Flutter Custom Painter & Canvas – Creating Custom UI Elements
February 28, 2025 | by Adesh Yadav
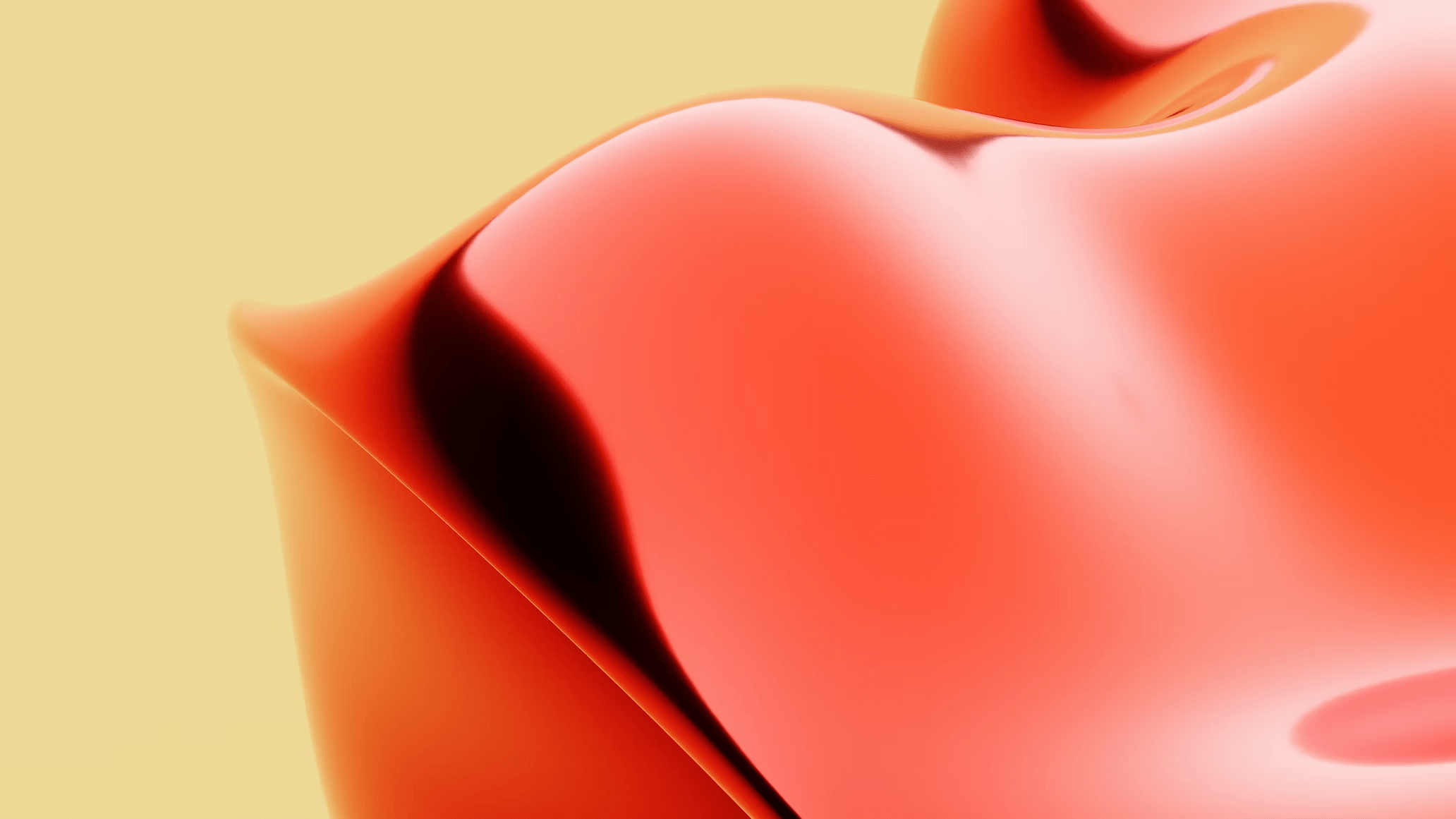
Flutter Custom painter & Canvas – Creating Custom UI Elements
In today’s mobile submission development landscape,crafting a unique user interface is crucial for standing out in a crowded market. Flutter offers a powerful solution for developers through its Custom Painter and Canvas classes. This article will guide you through the fundamentals of using Flutter’s Custom Painter to create stunning custom UI elements.
What is Flutter Custom painter?
The Custom Painter class in Flutter allows developers to implement custom graphics and shapes that go beyond the limitations of standard widgets.Leveraging the Canvas class,you can draw shapes,text,and images by defining a painting strategy. This is particularly useful for applications that require a standout design that cannot be achieved with standard graphical components.
Understanding the Canvas Class
the Canvas class provides low-level drawing operations, allowing you to:
- Draw shapes (rectangles, circles, lines, etc.)
- Fill and stroke paths
- Render text
- Manipulate individual pixels
Benefits of Using Flutter Custom Painter
The Custom Painter class brings several advantages, including:
- Versatility: Create bespoke designs tailored to your app’s needs.
- Performance: Render complex graphics efficiently with minimal overhead.
- Reusable Code: Build reusable custom widgets to streamline your development process.
Setting Up Custom Painter
Creating Your First Custom Painter
To get started, you need to create a class that extends the CustomPainter. Here’s a basic example:
dart
import ‘package:flutter/material.dart’;
class MyCustomPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
// Drawing a blue rectangle
final paint = Paint()..color = Colors.blue;
canvas.drawRect(Rect.fromLTWH(0, 0, size.width, size.height), paint);
// Drawing a red circle
paint.color = Colors.red;
canvas.drawCircle(Offset(size.width / 2, size.height / 2), size.width / 5, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) => false;
}
“`
To use this CustomPainter, wrap it in a CustomPaint widget:
“`dart
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: CustomPaint(
size: Size(200, 200),
painter: MyCustomPainter(),
),
),
);
}
“`
Advanced Techniques with Flutter Custom Painter
Using Paths for Complex Shapes
Paths can be used to create more complex shapes. You can define your paths using a Path object:
“`dart
@override
void paint(Canvas canvas, Size size) {
final paint = Paint()..color = Colors.green;
Path path = Path();
path.moveTo(size.width / 2, 0); // Start at the top center
path.lineTo(size.width, size.height); // Draw a line to the bottom right
path.lineTo(0, size.height); // Draw line to the bottom left
path.close(); // Close the path
canvas.drawPath(path, paint);
}
“`
Adding Gradients
Gradients can also be incorporated into your designs:
“`dart
@override
void paint(Canvas canvas, Size size) {
final Rect rect = Rect.fromLTWH(0, 0, size.width, size.height);
final Gradient gradient = LinearGradient(
colors:
);
final Paint paint = Paint()..shader = gradient.createShader(rect);
canvas.drawRect(rect, paint);
}
“`
Practical Tips for Using flutter custom Painter
Here are some practical tips to effectively utilize Custom Painter:
- Start Simple: Begin with basic shapes and gradually add complexity.
- Understand the Coordinate System: Familiarize yourself with Flutter’s coordinate system to draw accurately.
- Optimize Performance: Use shouldRepaint method smartly to prevent unneeded repaints.
Real-World Case Studies
Various applications have successfully leveraged custom Painter for innovative interfaces:
1. Graphic Data Visualization Apps
Many applications rely on Custom Painter to create dynamic charts or graphs, allowing for real-time data display.
2. Animated Custom UI
Custom Painter is also used to create eye-catching animations, as it gives developers full control over frame rendering.
conclusion
Flutter’s Custom Painter and Canvas classes offer developers powerful tools to create custom UI elements that stand out. With the ability to draw complex shapes, utilize paths, and implement gradients, the possibilities are virtually endless. Whether you are building a straightforward app or an intricate user interface, mastering these functionalities will significantly enhance the visual appeal of your projects. Now that you have an understanding of Flutter Custom Painter, it’s time to unleash your creativity and start designing unique UI elements!
“`
RELATED POSTS
View all