Flutter Form and TextFormField – Validation and Error Handling
March 8, 2025 | by Adesh Yadav
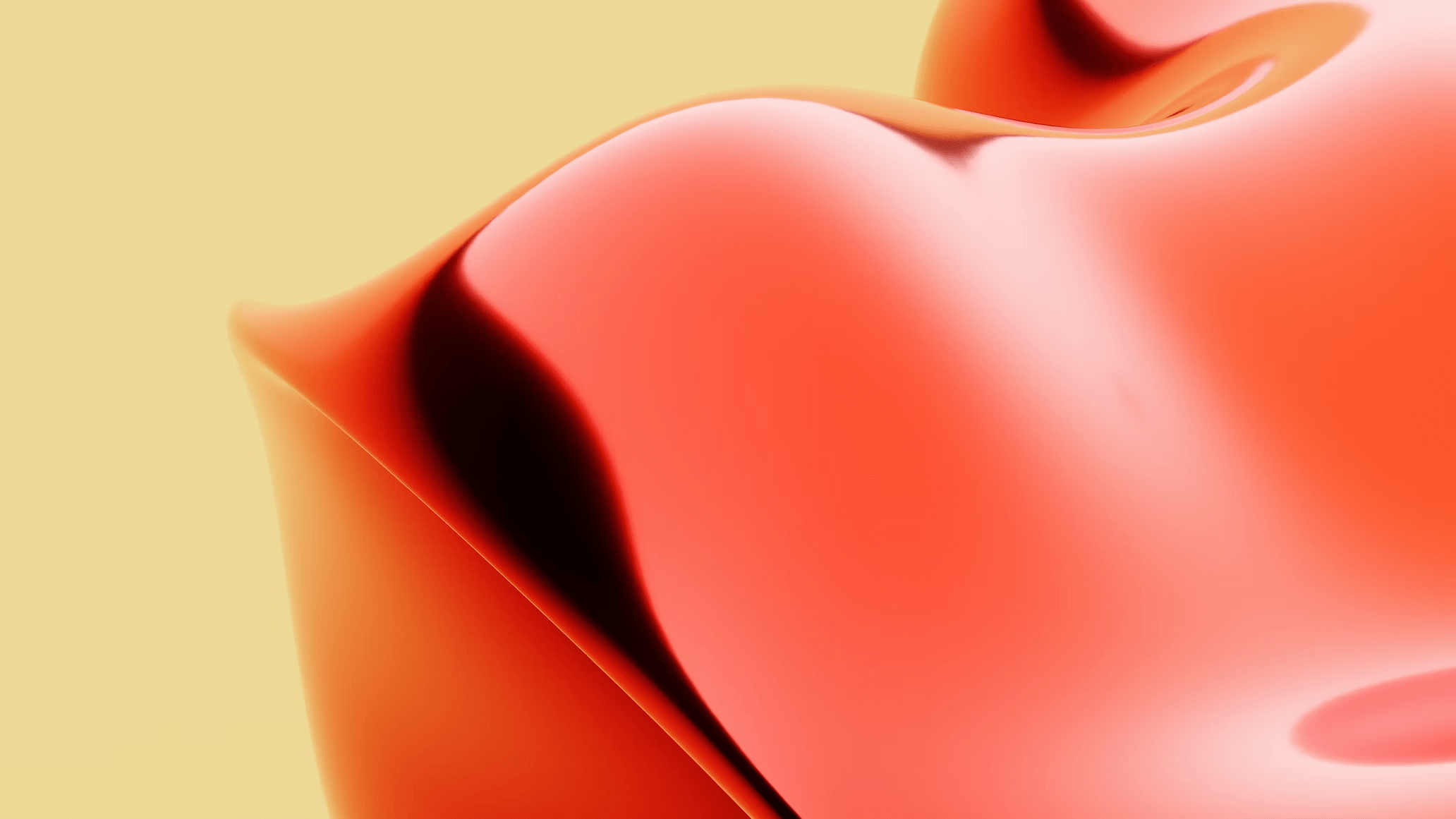
Flutter form and TextFormField – Validation and error Handling
In the realm of Flutter development, creating intuitive user interfaces is a top priority.User input forms are basic in gathering data, and ensuring that this data is valid is crucial. In this extensive guide, we’ll delve into Flutter’s Form and TextFormField widgets, focusing on validation and error handling. By the end of this article, you’ll be equipped with the knowledge to enhance user experiences in your apps.
Understanding Flutter Forms
Flutter offers a robust way to handle form data using the Form
widget. A Form in Flutter is a container for TextFormField
widgets and other input fields, which allows a systematic approach for validating user input.
Key Components of a Form
- Form: A parent widget that holds the form controls.
- TextFormField: A specialized input field for text input.
- GlobalKey: A key used to uniquely identify the Form and it’s state, enabling validation.
Implementing TextFormField
The TextFormField widget is an essential element for user input. It provides integrated validation features, making it a valuable addition to any form. Below is a simple implementation:
import 'package:flutter/material.dart';
class MyForm extends StatefulWidget {
@override
_MyFormState createState() => _MyFormState();
}
class _MyFormState extends State {
final _formKey = GlobalKey();
String _name;
@override
Widget build(BuildContext context) {
return Form(
key: _formKey,
child: Column(
children: [
TextFormField(
decoration: InputDecoration(labelText: 'Enter your name'),
validator: (value) {
if (value.isEmpty) {
return 'Please enter some text';
}
return null;
},
onSaved: (value) {
_name = value;
},
),
ElevatedButton(
onPressed: () {
if (_formKey.currentState.validate()) {
_formKey.currentState.save();
// Process the data.
}
},
child: Text('Submit'),
),
],
),
);
}
}
Validation in Flutter Forms
Validation is essential to ensure data integrity. Flutter allows you to define validation rules within the validator
property of a TextFormField
. This property takes a function that returns a string, which represents the error message.
Common Validation Techniques
- Required Fields: ensure that fields are not left empty.
- Pattern Matching: Use Regular Expressions to match specific patterns,such as email addresses.
- Range Validation: Restrict numerical input to a specific range.
Example: Email Validation
validator: (value) {
if (value.isEmpty) {
return 'Please enter an email';
}
const pattern = r'^[^@]+@[^@]+.[^@]+';
final regExp = RegExp(pattern);
if (!regExp.hasMatch(value)) {
return 'Please enter a valid email';
}
return null;
},
Error Handling Strategies
Effective error handling not only improves user experience but also boosts your application’s reliability. Flutter provides various ways to handle errors:
Displaying Error Messages
To show error messages,guide users to correct their input. This can be accomplished easily with Flutter’s built-in functionality.
TextFormField(
decoration: InputDecoration(
labelText: 'Email',
errorText: _errorMessage, // Dynamically set during validation
),
);
Focus management
When a validation error occurs, it helps to focus the user on the erroneous field:
FocusScope.of(context).requestFocus(focusNode);
benefits of Using Flutter Forms
- Integration: Easy integration with backend services for data submission.
- Built-in Validation: Save development time with built-in validation capabilities.
- Customization: Tailor form fields to match user experience and design needs.
Best Practices for Form Validation
- Validate Inputs Upon Submission: Ensure all fields are checked when the form is submitted.
- Enable Input Feedback: Show user-friendly error messages next to the respective input fields.
- Keep the UI Clean: Avoid cluttering the form with too manny fields or validation messages.
Case Study: Real-World application of Flutter Forms
Consider a shopping application where users are required to fill out their shipping information. Implementing a well-structured form with TextFormField validation ensures that:
- Users provide accurate information.
- Invalid entries are caught before accessing payment screens.
- Improved user satisfaction through instant feedback on errors.
First-Hand Experience: My Journey with Flutter Forms
As a developer,I found that utilizing TextFormField for my project’s forms simplified the data gathering process. Initially, I underestimated the power of built-in validation. However, upon incorporating it, I witnessed a significant decrease in user input errors and an increase in form completion rates.
Conclusion
Mastering flutter’s Form and TextFormField for validation and error handling is imperative for any app developer. By implementing structured forms with robust validation, you not only improve user experience but also ensure data integrity.Armed with these insights and best practices, you’re ready to enhance the forms in your Flutter applications. Start building better forms today!
RELATED POSTS
View all