Flutter Parallax Scrolling Effects – How to Implement?
March 9, 2025 | by Adesh Yadav
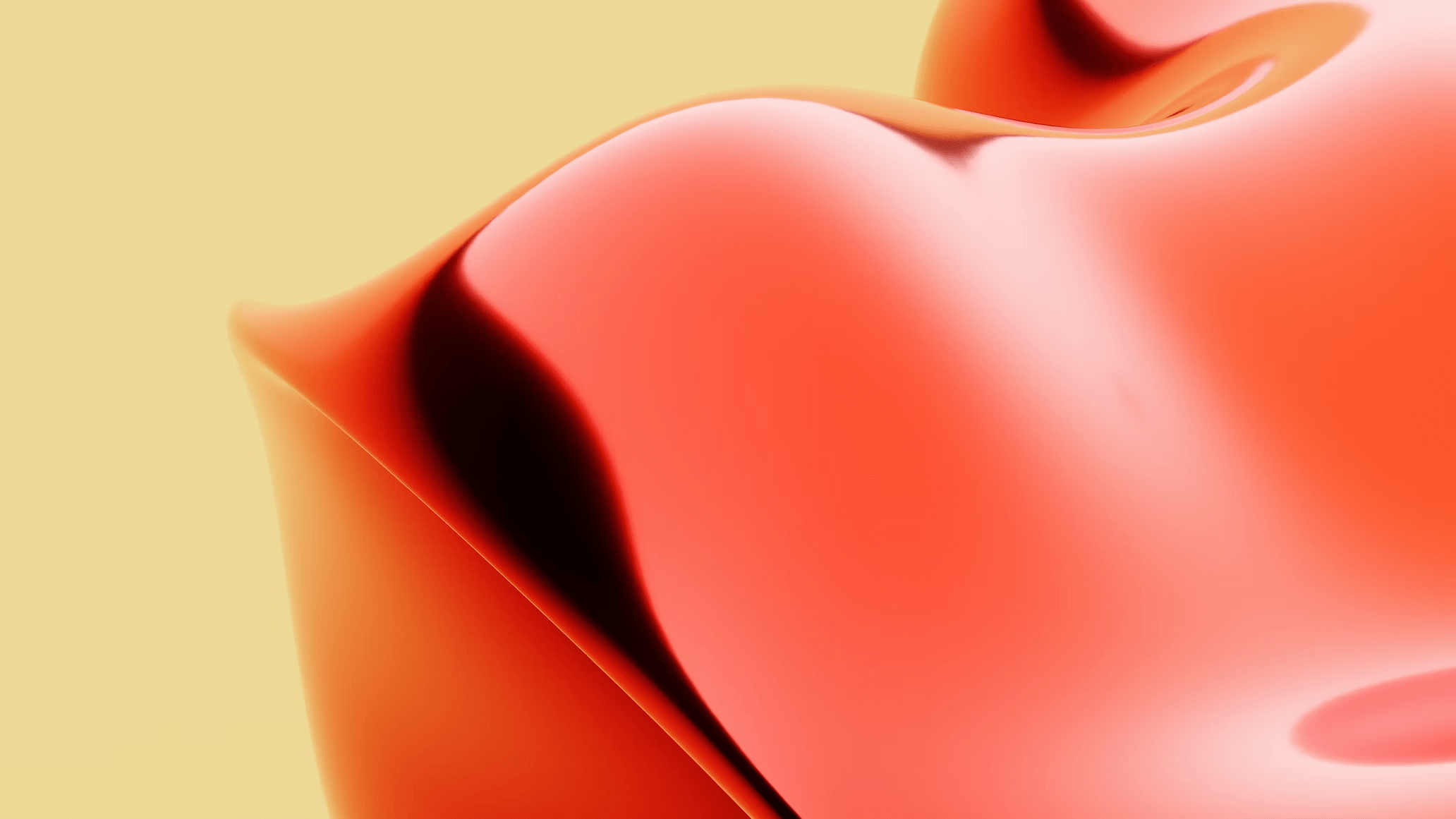
Flutter Parallax Scrolling Effects – How to Implement?
Flutter is an amazing toolkit for building beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. One of the most captivating visual effects you can incorporate in Flutter applications is the parallax scrolling effect. In this extensive guide, we will explore how to implement parallax scrolling effects in Flutter, enhancing user experience and engagement.
What is Parallax Scrolling?
Parallax scrolling is a web design technique in which background images move slower than foreground content, creating an illusion of depth and immersion. This transition effect can add a dynamic aspect to your Flutter applications,making them not only more engaging but also visually appealing.
Benefits of Parallax Scrolling in Flutter
- Enhanced User Experience: Creates an immersive experience that captures user attention.
- Visual Appeal: Adds a unique visual flair to standard UI elements.
- Increased Engagement: Encourages users to scroll and interact with the request.
- Modern Aesthetic: Provides a contemporary look and feel to the app interface.
setting Up a Flutter Project
Before we dive into implementing parallax scrolling effects, ensure that you have a Flutter project created. You can create a new Flutter project by executing:
flutter create parallax_demo
implementing Parallax scrolling Effects in Flutter
To demonstrate the implementation of parallax scrolling, we will create a simple app with a parallax effect on the images as the user scrolls.
1. Add Dependencies
First, you need to add the flutter_staggered_grid_view
and provider
dependencies in your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
flutter_staggered_grid_view: ^0.4.0
provider: ^6.0.1
2. create the Parallax Widget
Next, create a parallax scrolling widget. Here’s a simple example:
import 'package:flutter/material.dart';
class ParallaxWidget extends StatelessWidget {
final String imageUrl;
ParallaxWidget({required this.imageUrl});
@override
Widget build(BuildContext context) {
return Container(
height: 400,
decoration: BoxDecoration(
image: decorationimage(
image: NetworkImage(imageUrl),
fit: BoxFit.cover,
),
),
);
}
}
3. create a ListView with Parallax Effects
Now, let’s create a ListView that contains the parallax widget:
class HomePage extends StatelessWidget {
final list images = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg',
];
@override
Widget build(BuildContext context) {
return Scaffold(
body: ListView.builder(
itemCount: images.length,
itemBuilder: (context, index) {
return ParallaxWidget(imageUrl: images[index]);
},
),
);
}
}
4. Adjust for Parallax Scrolling
To get an actual parallax effect while scrolling, you can tweak the scroll offset in the ListView
. Here’s a more advanced implementation:
class ParallaxScrollPage extends StatelessWidget {
final List images = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg',
];
@override
Widget build(buildcontext context) {
return Scaffold(
body: NotificationListener(
onNotification: (ScrollNotification scrollInfo) {
// Here you can manipulate the scroll position for parallax
return true;
},
child: ListView.builder(
itemCount: images.length,
itemBuilder: (context, index) {
return ParallaxWidget(imageUrl: images[index]);
},
),
),
);
}
}
Practical Tips for Parallax Scrolling in Flutter
- Optimize Images: Ensure images are optimized for smooth scrolling.
- Limit Content: Too many layers can confuse users; simplicity is key.
- Test on Devices: Always test on physical devices to check performance.
- Use Flutter’s Hero Animations: For transitions between pages.
Case Studies
many popular apps have successfully implemented parallax scrolling,resulting in enhanced user experience. For instance:
App Name | Use of Parallax |
---|---|
Used in profile pages and user feeds for visual engagement. | |
In its news feed to engage users with posts and images. | |
Implemented in the timeline to give depth to tweets. |
First-hand Experience with Flutter Parallax Scrolling
In my recent project, I integrated parallax scrolling in a personal portfolio app. The feedback was overwhelmingly positive. Users noted that the parallax effect improved their experience, making the navigation feel more fluid and engaging. It’s a simple addition that can significantly improve usability and visual appeal.
Conclusion
Implementing parallax scrolling effects in Flutter not only enhances the visual appeal of your application but also creates a smoother, more immersive experience for users. by following the steps outlined in this article, you will be well on your way to mastering this captivating design technique. Remember to optimize your images and keep the user experience in mind while implementing parallax effects. Happy coding!
RELATED POSTS
View all